json.decoder.jsondecodeerror: expecting value: line 1 column 1 (char 0) How to Solve
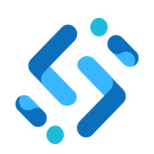
2024-08-21 on Engineering
7 min read
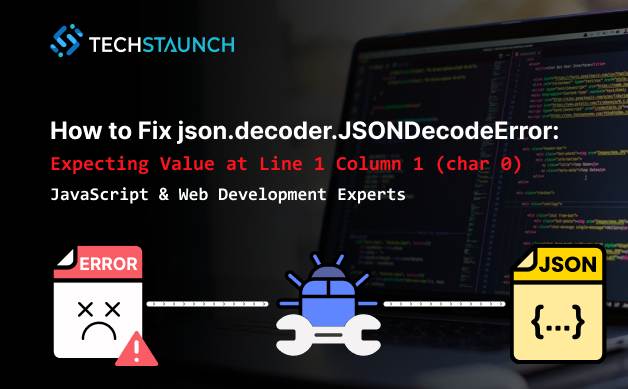
Overview of JSON and its Role in Programming
JavaScript Object Notation (JSON) is a lightweight data exchange format often used in web development. It is supposed to be used as a medium for transmitting structured and easily understandable data from the server to the client. JSON comprises key-value pairs in texts, making it simple to use with JavaScript and many other programming languages. Consequently, it is widely used in APIs and web services that help facilitate inter-platform and inter-device communication.
Let us look at an example; when you request a server as a client, typically, it will respond with data formatted as JSON. Programmers then read this information from the JSON file they get only what they need before using it on their applications. This simplicity and interoperability are what have made JSON so popular.
Nevertheless, failure to handle JSON correctly or encountering unexpected contents sometimes leads to errors during working with JSON. json.decoder.JSONDecodeError is one of the most common mistakes users make while using this format.
Explanation of the json.decoder.JSONDecodeError
Python json. decoder.JSONDecodeError commonly happens when one tries to decode a JSON object in Python and the input data is not in valid JSON format. It specifically means that “json.decoder.JSONDecodeError: Expecting value: line 1 column 1 (char 0)” which appears as an error message indicates that your program attempts to parse a JSON string but finds nothing or invalid content right at the beginning.
This error means that the needed information is missing or wrongly organized. The error tells us that the decoder anticipated an input starting with a value but could not find any. This can happen because of several reasons, which we will now look into in this section.
Causes of the JSON decoder.JSONDecodeError
Empty JSON String
One of the most common causes of this error is trying to decode a JSON string that is empty. For example, if an API returns nothing or the supposed JSON data file is blank, then when you try to parse it, the JSON. decoder.JSONDecodeError exception will be thrown. JSON expects valid syntax but an empty string can’t fulfill that condition.
import json
response = "" # Empty string instead of valid JSON
data = json.loads(response) # Raises JSONDecodeError
In this scenario, the program attempts to load the empty string as JSON, which results in the error.
Incorrectly Formatted JSON
Other common causes are JSON data that is not correctly formatted. JSON decoding problems can also be caused by tiny mistake like a missing curly bracket or an unquoted key. Therefore, if the syntax does not follow what JSON expects it to be as it is strict about its format, then, there will be an error during parsing.
import json
response = "{'key': 'value'}" # Incorrect JSON format (single quotes instead of double)
data = json.loads(response) # Raises JSONDecodeError
The correct JSON format should use double quotes for keys and values. Incorrect formatting is a frequent cause of the json.decoder.JSONDecodeError.
Network or API Issues
Sometimes the error happens because of network and API issues. To clarify, whenever the server cannot respond with proper feedback due to connectivity difficulties when making an API call, then the client could receive either an incomplete or empty payload. This invalid answer referred to as "json.decoder.JSONDecodeError,” might be obtained after correctly performing your request to any particular website.
import requests
import json
response = requests.get("https://api.example.com/data")
data = json.loads(response.text) # Raises JSONDecodeError if the API response is empty or malformed
This could be caused by server-side issues, poor network conditions, or unexpected response formats
File I/O Errors
Errors in file handling can also sometimes result in json.decoder.JSONDecodeError. If a program attempts to load a JSON object from a corrupted or empty file then its decoder will fail on parsing the content causing this error.
import json
with open("data.json") as f:
data = json.load(f) # Raises JSONDecodeError if the file is empty or contains invalid JSON
These file I/O errors can occur due to file corruption, incorrect file paths, or empty files.
Troubleshooting the Error
Diagnosing the Issue by Checking the JSON Source
When faced with an error json. decoder.JSONDecodeError, first look at where the JSON data is coming from. If it is a JSON from API response, check the actual response content. If it comes from a file, open it and make sure that it has correct JSON format. Find out its source to identify exactly what caused the error.
This can be done using tools like Postman for API responses or manually opening JSON files in a text editor.
Handling Empty JSON Responses
In order to handle empty JSON responses, you should add checks before you try parsing the data. For those working with APIs, always ensure that the decoder receives valid JSON as input.
import json
response = "" # Simulating an empty response
if response:
data = json.loads(response)
else:
print("Empty response, skipping decoding.")
This simple check prevents the program from trying to decode an empty string, avoiding the JSONDecodeError.
Ensuring Correct JSON Formatting
It is important to ensure that the correct formatting of JSON is followed before parsing is done. Validate structure of the JSON data to confirm if it follows the expected pattern. You may utilize jsonlint.com and other such utilities which will quickly tell you whether your JSON is properly formatted or not.
import json
response = '{"key": "value"}' # Correctly formatted JSON
data = json.loads(response) # Successful decoding
Preventive Measures
Validating JSON Before Decoding
Always check the structure of incoming data before decoding JSON. For instance, when dealing with API supplied JSON, verify that the response is a valid JSON before attempting to parse it. This can be done using simple if-else conditions or by catching potential exceptions that might be raised during decoding.
Implementing Robust Error Handling
Implementing robust error handling ensures your application responds gracefully in cases where JSON decoding could fail. Employ try-except blocks to catch and handle the JSONDecodeError accordingly.
import json
try:
data = json.loads(response)
except json.decoder.JSONDecodeError:
print("Failed to decode JSON. Please check the data source.")
This approach prevents the application from crashing and allows you to take corrective action.
Advanced Considerations
Customizing JSON Decoding Behavior
Occasionally, there may be a requirement for you to tailor JSON decoding behavior in your program. Python’s json module enables the user to extend/alter its behavior as required. For instance, you may include customized error handling for specific JSON errors or improve JSON parsing capabilities using third-party libraries.
Exploring Alternatives to JSON
JSON is a popular data interchange format but not the only one out there. There are other formats such as XML, YAML, and Protocol Buffers (Protobuf) which can provide users with different benefits depending on their unique requirements. Structured data heavy environments or scenarios requiring high performance might be better off considering these options as opposed to JSON.
Conclusion
Recap of Key Points and Final Tips
Common issue arising when an attempt is made towards interpreting an invalid or empty JSON data is “json. decoder.JSONDecodeError: Expecting value: line 1 column 1 (char 0).” To resolve this problem, it is necessary to understand its causes; whether it is blank text or wrong formatting; network problems or file I/O errors.
In conclusion always validate your Json before attempting to decode it and put in place stringent error handling mechanism that will help catch any issues early enough. This way your application will be able to handle JSON data reliably without falling into common pitfalls.
Check out our other related blogs for more insights into industry trends:
Can You Stop a forEach Loop in JavaScript? - Interview Insights
Programming Securely with WebKit: Best Practices for Web Developers