Programming Securely with WebKit: Best Practices for Web Developers
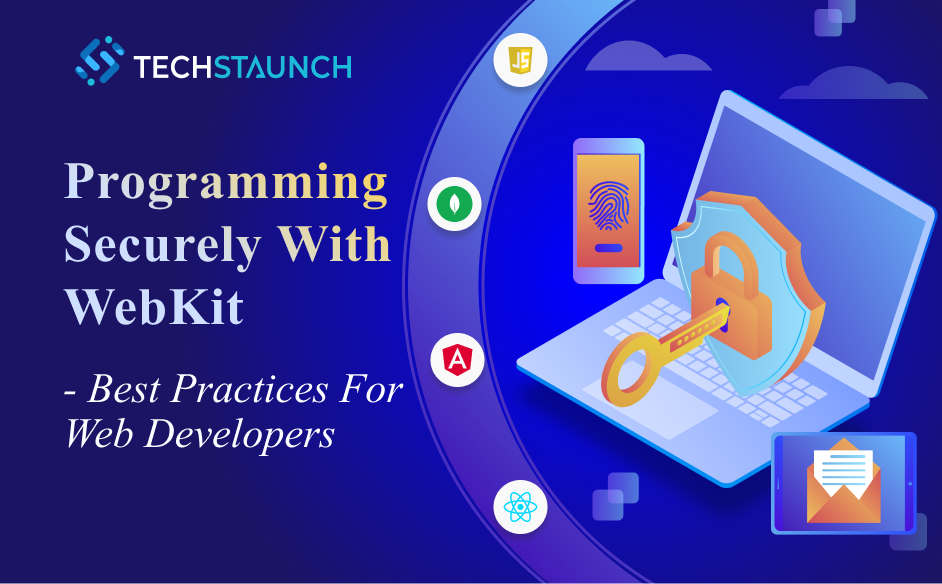
Introduction
With the continuous development of web applications, ensuring their security
has become increasingly important. The use of WebKit as a web-development
framework by developers worldwide has created unique threats that require
appropriate mitigation. In this blog, we will explore the essential best practices
that modern web developers can implement to protect sensitive data and
applications. We will be looking into the latest research and trends to provide
practical insights into the most recent security threats and practices for secure
programming with WebKit.
Understanding WebKit's Architecture
WebKit is an open-source web browser engine that plays a crucial role in how content is rendered on the web. It consists of several key components:
WebCore:
The main rendering engine responsible for parsing HTML and CSS, layout, rendering and scripting.
JavaScriptCore:
The JavaScript engine used to execute scripts efficiently.
WebKit API:
Facilitates interaction between WebCore and the host application, ensuring seamless communication and processing.
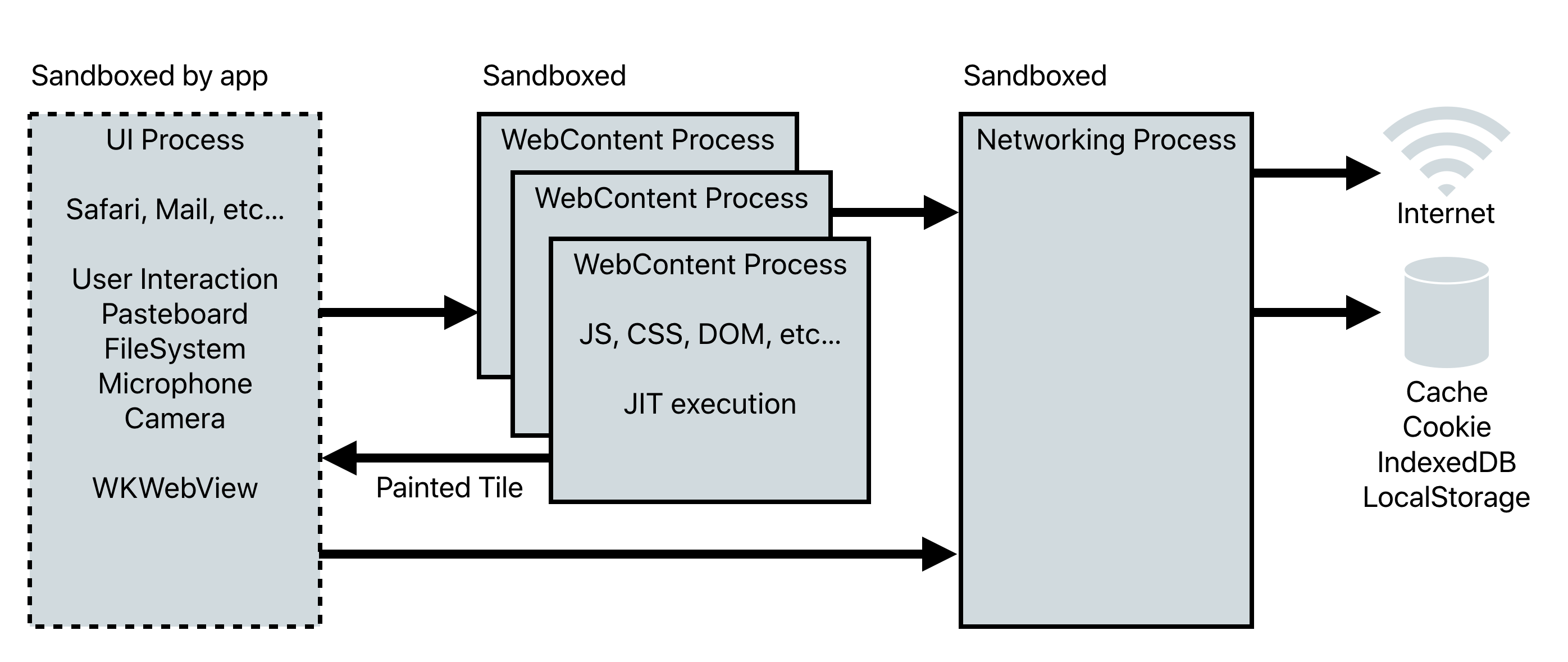
Key Security Concerns with WebKit
Like any complex system, WebKit is susceptible to security vulnerabilities, which can be exploited by malicious entities. Some of the most common vulnerabilities include:
Cross-Site Scripting (XSS):
Where attackers inject malicious scripts into content from otherwise trusted websites.
Cross-Site Request Forgery (CSRF):
Where unauthorized commands are transmitted from a user that the web application trusts.
Security Misconfiguration:
Often seen in improper handling of CORS (Cross-Origin Resource Sharing) settings that could allow unwanted cross-origin interactions.
Addressing these vulnerabilities requires a thorough understanding of WebKit's security capabilities and the implementation of specific security practices.
Implementing Origin Checks
Origin checks are a fundamental aspect of web security. They ensure that the content or scripts interact as intended only with the sources from which they are originated. In WebKit, you can enforce strict origin policies by:
Setting Content Security Policies (CSP):
Specify which origins are trustworthy when loading content, thus preventing XSS attacks.
Validating Document Origins:
Ensure that scripts can only access data from the same origin, or a specifically allowed one, reducing the risk of CSRF attacks.
Example Code Snippet:
// Enforcing CSP with meta tag
const csp = "default-src 'self'; script-src 'none';"; document.createElement("meta").setAttribute("http-equiv", "Content-Security-
Policy");
document.createElement("meta").setAttribute("content", csp);
document.getElementsByTagName("head")[0].appendChild(meta);
Ensuring Message Validation:
When components within WebKit communicate, it is vital to validate these messages. Ensuring the integrity and security of inter-component communication prevents malicious data from being processed. Implement
message validation by:
Verifying Message Sources:
Check the origin of incoming messages to ensure they come from trusted sources.
Sanitizing Input:
Always sanitize input to avoid executing malicious content, especially when the input comes from external sources.
Practical Example:
window.addEventListener("message", (event) =>
{
if (event.origin !== "https://trusted-origin.com")
return;
// Process the message safely after validation
});
Secure Communication Protocols:
To enhance security further, implementing secure communication protocols like HTTPS is crucial. Configuring WebKit to enforce these protocols involves:
Using Secure Cookies: Set cookies with the Secure attribute to ensure they are sent over HTTPS only.
Enforcing HTTPS: Redirect all HTTP requests to HTTPS using HSTS (HTTP
Strict Transport Security) headers.
Case Studies:
To fully appreciate the significance of robust security practices within WebKit, examining past security breaches can be enlightening. Here are a couple of instances where lack of secure programming led to significant vulnerabilities:
Case Study 1:
XSS Vulnerability in WebKit-Based Browser: A prominent incident involved an XSS attack that exploited a flaw in the way WebKit handled cross-origin resource sharing. Attackers injected malicious scripts into ads displayed on reputable sites, which then executed on the user's browser, compromising personal data.
Lesson Learned:
This breach underscored the critical need for stringent CSPs and the necessity of sanitizing all inputs, especially those originating from third-party sources.
Case Study 2:
CSRF Attack Exploiting Cookie Mismanagement: Another case involved CSRF attacks where attackers hijacked user sessions by exploiting WebKit's cookie management. The browser failed to restrict cookie access to HTTPS connections only, allowing attackers to intercept session cookies over unsecure connections.
Lesson Learned:
This highlighted the importance of marking cookies as Secure to ensure they are transmitted only over HTTPS and implementing HSTS to prevent protocol downgrade attacks.
Tools and Resources
To assist developers in implementing and maintaining security measures within WebKit, several tools and resources are available:
WebKit Inspector:
Use this tool for debugging and testing the security of your web applications directly within the browser environment.
OWASP ZAP:
An open-source security tool that helps identify security vulnerabilities in your applications during development and testing.
SSL Labs' SSL Test:
A tool to evaluate the security configuration of your HTTPS servers.
By leveraging these tools, developers can gain insights into potential security flaws in their applications and take corrective actions proactively.
Final Thoughts and Best Practices Recap
As we wrap up this discussion on securing WebKit, it’s crucial to iterate some of the best practices that every web developer should follow:
Implement and strictly enforce CSPs:
To limit the sources of executable scripts and protect against XSS attacks.
Validate all origins and messages:
Rigorously to prevent CSRF and other inter-component communication attacks.
Adopt HTTPS exclusively:
And configure security features like Secure cookies and HSTS to safeguard data in transit.
By adopting these practices, developers can significantly bolster the security of their web applications, providing a safer environment for users and protecting sensitive data from potential threats.
Call to Action
Don’t wait for a breach to occur. Proactively audit your WebKit applications regularly, update your security practices as needed, and continuously educate yourself and your team on the latest in web security. The safety of your applications—and the trust of your users—depends on it.
Check out our other related blogs for more insights into industry trends:
Can You Stop a forEach Loop in JavaScript? - Interview Insights
Celebrating Our Tech Journey: Highlights from 2023 | TechStaunch
Web Development Trends 2024: Innovations Redefining the Digital Landscape | TechStaunch
Loading...