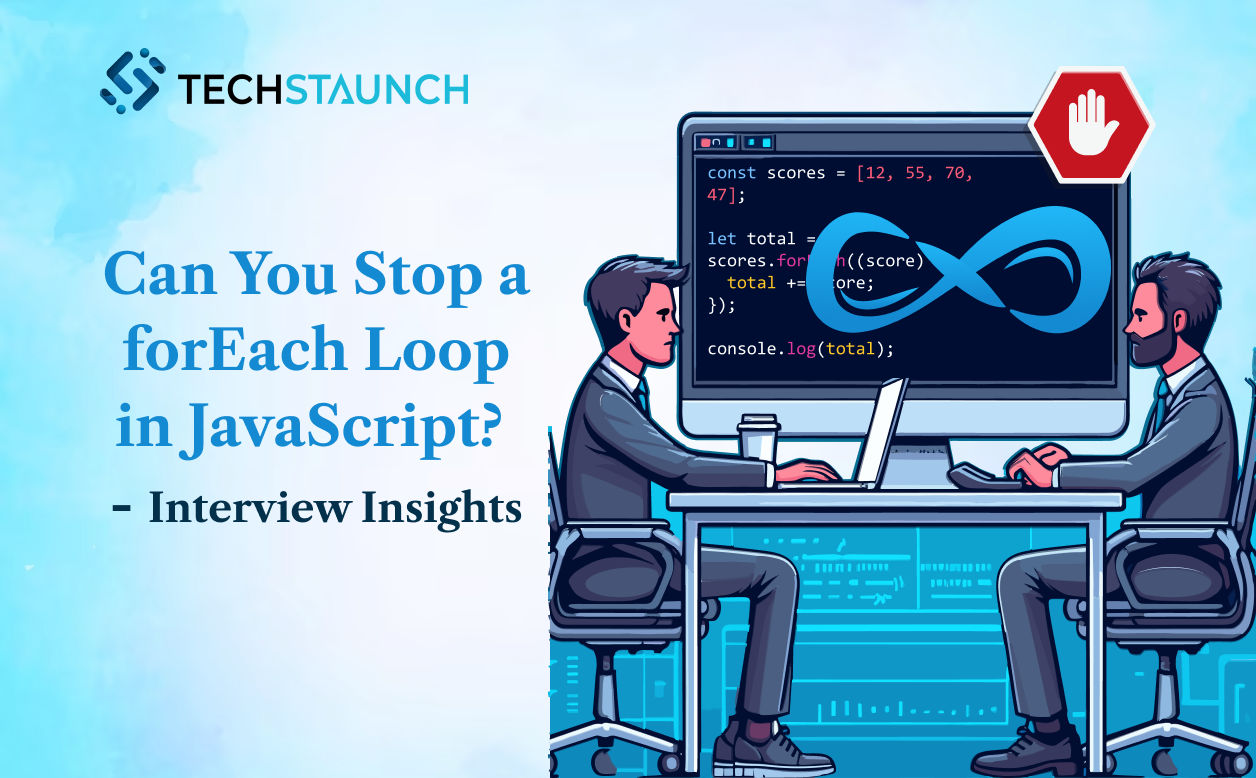
Understanding "forEach" in JavaScript
The forEach method in JavaScript is a part of the Array prototype. It allows developers to execute a function on each element in an array. For example:
javascript
1const numbers = [1, 2, 3,4,5];
2numbers.forEach(number => {
3console.log(number);
4});
This code logs each number in the numbers array. Unlike other loop methods, forEach is simple and concise, making it a favorite among developers. However, it comes with a limitation – it cannot be stopped or broken out of once it starts.
Can You Stop "forEach" ?
Direct Answer: No, you cannot stop a forEach loop once it begins. The forEach method executes the provided function for each array element until the end of the array is reached. This lack of a 'break' mechanism is by design.
Common Misconceptions: Some developers assume that throwing an exception can stop forEach. While it does halt execution, it's not a recommended practice as it disrupts the program flow and can lead to unmanageable code.
Alternatives: For scenarios where you need to stop or break a loop, other methods like for, for...of, Array.prototype.some(), and Array.prototype.every() are more suitable. These methods provide more control over the iteration process.
When to Use "forEach" vs Alternatives
forEach is ideal for scenarios where you need to apply a function to every element in an array and when there's no need to stop or break the loop. On the other hand, if you need more control over the iteration, like stopping based on a condition, for or for...of loops are preferable. The some and every methods are also great for conditions-based iterations.
Interview Questions Related to "forEach"
In interviews, you might encounter questions like:
- "Explain the forEach method in JavaScript and its limitations."
- "How would you iterate over an array and stop based on a condition in JavaScript?"
The key to answering these questions is not just to provide the direct answer but also to demonstrate an understanding of when and why to use forEach and its alternatives.
Read More: 10 Microservices Anti-Patterns, Top Backend Node Interview Questions 2024
Conclusion
Understanding the forEach methods in JavaScript and its inability to be stopped is crucial for developers, especially in interview scenarios. While forEach offers simplicity, it's essential to know when to use other looping methods for more control. This knowledge not only showcases your technical skills but also your ability to choose the right tool for the task.
FAQs
To find the maximum value in an array using a for loop, start by assuming the first element is the max, then loop through each element, comparing it to the current max, and update the max if a larger value is found. For the minimum value, do the same but update for smaller values.
// for loop
for (let i = 0; i < 5; i++) {
console.log(i); // 0, 1, 2, 3, 4
}
// for-in loop: Iterates over the enumerable properties of an object, providing access to the keys (property names)
const obj = { a: 1, b: 2 };
for (let key in obj) {
console.log(key); // 'a', 'b'
}
// for-of loop: Iterates over iterable objects (like arrays, strings) and provides access to their values.
// It's part of ES6 and cannot be used directly with objects unless they implement the iterable protocol
const arr = [10, 20, 30];
for (let value of arr) {
console.log(value); // 10, 20, 30
}
TLDR Summary
This blog delves into the intriguing question often posed in interviews: "Can you stop forEach in JavaScript?" The straightforward answer is no, and the blog explains why, detailing the limitations of forEach. It also explores various alternatives like for, for...of, some, and every loops, which provide more control over iterations. Understanding these differences is not only crucial for acing technical interviews but also for effective JavaScript programming.
Check out our other related blogs for more insights into industry trends:
Celebrating Our Tech Journey: Highlights from 2023 | TechStaunch
Optimizing Your Web Store for the Holiday Rush: A Technical Guide
Web Development Trends 2024: Innovations Redefining the Digital Landscape | TechStaunch