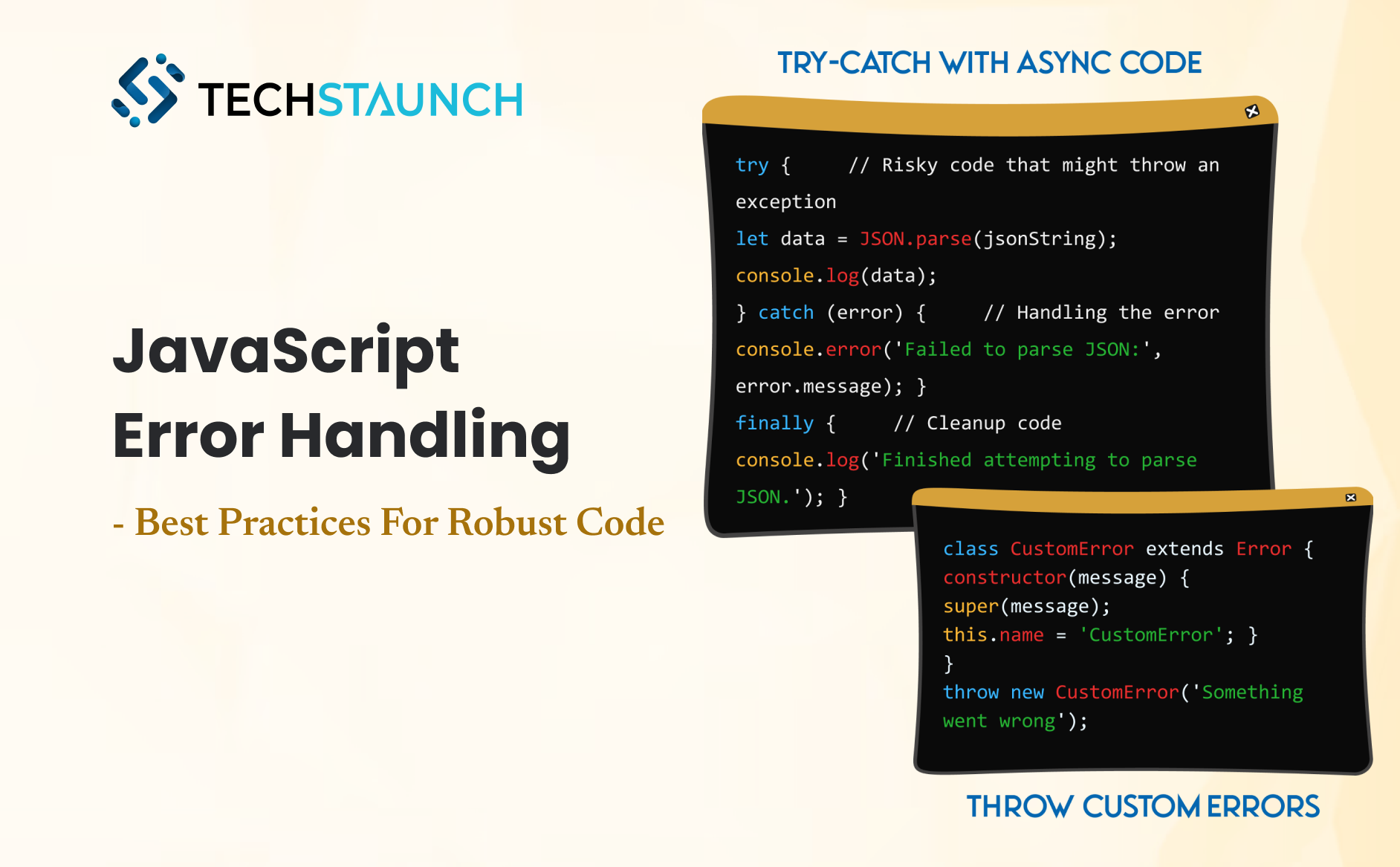
Introduction
Error handling is a crucial aspect of robust software development. In JavaScript, effective error handling ensures that your applications are reliable, maintainable, and user-friendly. This blog will guide you through the fundamentals of error handling in JavaScript, differentiate between handling errors in synchronous and asynchronous code, and provide strategies for managing asynchronous errors. Finally, we will explore best practices for building resilient applications.
Read More : JavaScript: How to Check if a Key Exists in an Object
Error Handling Fundamentals
Overview of Basic Error Handling Techniques in JavaScript
Error handling in JavaScript can be broadly categorized into two types: synchronous and asynchronous error handling.
- try...catch Statement:
The try...catch statement is used to handle exceptions that occur during the execution of a block of code. It allows you to catch errors and handle them gracefully without crashing the entire application.
javascript
1try { 2 // Code that may throw an error 3 let result = riskyOperation(); 4 console.log(result); 5} catch (error) { 6 // Code to handle the error 7 console.error('An error occurred:', error); 8} finally { 9 // Code that will run regardless of an error occurring 10 console.log('Operation completed'); 11}
- Throwing Errors:
You can explicitly throw errors using the throw statement. This is useful for creating custom error messages and handling specific conditions.
javascript
1function divide(a, b) {
2 if (b === 0) {
3 throw new Error('Division by zero is not allowed');
4 }
5 return a / b;
6}
7try {
8 let result = divide(10, 0);
9 console.log(result);
10} catch (error) {
11 console.error('An error occurred:', error.message);
12}
Synchronous vs. Asynchronous Errors
Differentiating Between Synchronous and Asynchronous Errors
Understanding the difference between synchronous and asynchronous errors is essential for effective error handling.
- Synchronous Errors:
Synchronous errors occur during the execution of a block of code and are caught immediately. These errors can be handled using try...catch.
javascript
1try { 2 let result = JSON.parse('invalid JSON string'); 3} catch (error) { 4 console.error('Parsing error:', error.message); 5}
- Asynchronous Errors:
Asynchronous errors occur in code that runs asynchronously, such as callbacks, Promises, and async/await. These errors cannot be caught directly by try...catch since the asynchronous code is executed outside the synchronous block. Consider the following example with a setTimeout function:
javascript
1try {
2 setTimeout(() => {
3 throw new Error('Asynchronous error');
4 }, 1000);
5} catch (error) {
6 console.error('Error caught:', error.message);
7}
The try...catch block will not catch the error because the setTimeout function runs asynchronously. To handle such errors, different strategies are needed.
Strategies for Asynchronous Error Handling
Callbacks
Error handling in callbacks is done by passing an error object as the first argument to the callback function. This pattern is known as the error-first callback pattern.
javascript
Promises
Promises provide a cleaner way to handle asynchronous operations. They can be in one of three states: pending, fulfilled, or rejected. Error handling is done using the .catch method.
javascript
Async/Await
The async/await syntax provides a more straightforward way to work with Promises. It allows you to write asynchronous code that looks synchronous, making it easier to read and maintain. Error handling is done using:
javascript
Building Resilient Applications - Tips and Best Practices
Logging
Implement comprehensive logging to capture error details. This helps in diagnosing issues and understanding application behavior.
javascript
1function logError(error) {
2 console.error('Timestamp:', new Date().toISOString());
3 console.error('Error Message:', error.message);
4 console.error('Stack Trace:', error.stack);
5}
6try {
7 // Simulate error
8 throw new Error('Sample error');
9} catch (error) {
10 logError(error);
11}
Monitoring
Use monitoring tools like Sentry, LogRocket, or New Relic to track errors in real-time. These tools provide insights into error occurrences and their impact on users.
Graceful Degradation
Design your application to handle errors gracefully. Ensure that critical functionalities remain available even when parts of the application fail.
javascript
Testing
Implement thorough testing to identify and fix errors early in the development process. Use unit, integration, and end-to-end tests to cover various scenarios.
javascript
1const assert = require('assert');
2function divide(a, b) {
3 if (b === 0) {
4 throw new Error('Division by zero is not allowed');
5 }
6 return a / b;
7}
8
9try {
10 assert.strictEqual(divide(10, 2), 5);
11 assert.strictEqual(divide(10, 0), 'Error');
12} catch (error) {
13 console.error('Test failed:', error.message);
14}
Conclusion
Effective error handling is essential for building robust JavaScript applications. By understanding the differences between synchronous and asynchronous errors and implementing appropriate error-handling strategies, you can ensure that your applications are reliable and user-friendly. Utilize logging, monitoring, graceful degradation, and testing to further enhance the resilience of your applications.
Incorporate these best practices into your development workflow to create error-tolerant and maintainable JavaScript applications. Happy coding!
Check out our other related blogs for more insights into industry trends:
Can You Stop a forEach Loop in JavaScript? - Interview Insights
Programming Securely with WebKit: Best Practices for Web Developers
Web Development Trends 2024: Innovations Redefining the Digital Landscape | TechStaunch