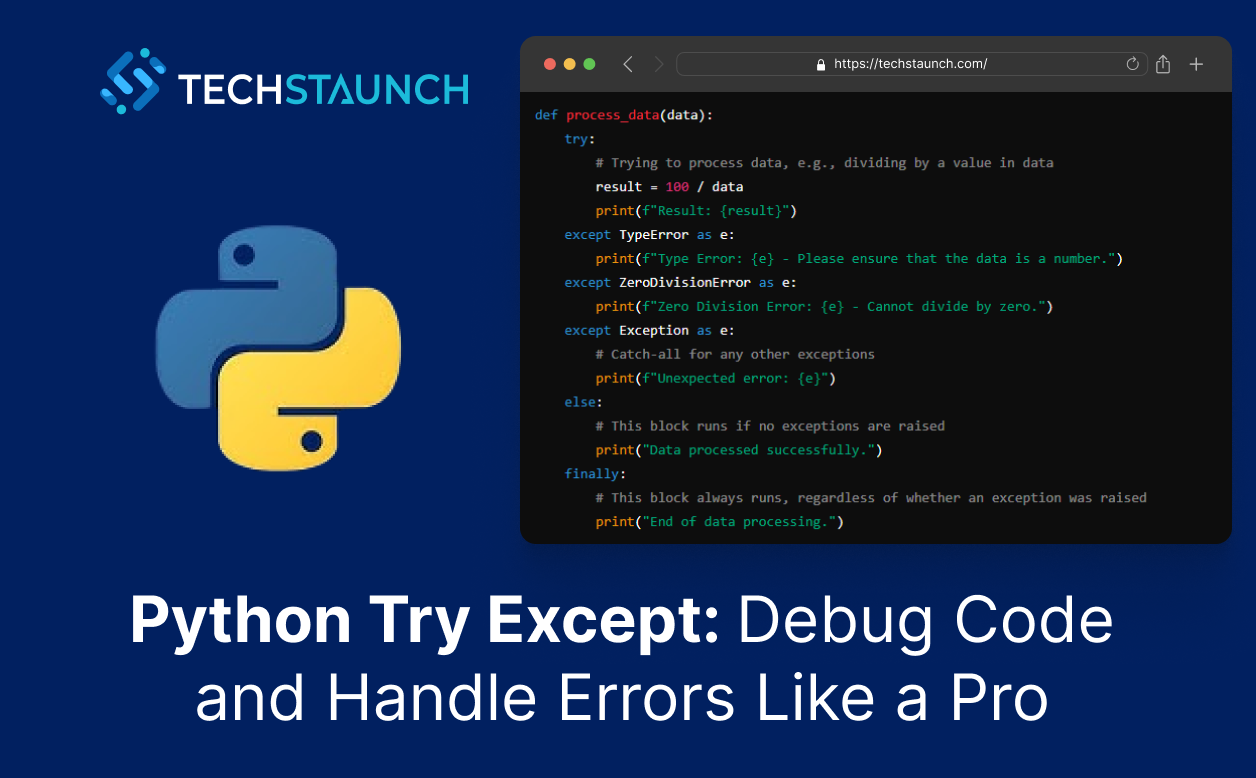
Introduction
Ever had your Python program crash unexpectedly? Error handling is your shield against such surprises. Whether you're building a simple script or a complex application, mastering Python's try-except is crucial for writing robust, reliable code.
What is Error Handling, and Why Is It Important?
In programming, errors are inevitable. From simple typos to complex runtime errors, they can disrupt your program's flow. Error handling is your way of gracefully managing these situations.
Key Benefits
- Prevent sudden program crashes
- Improve user experience
- Simplify debugging
- Enhance code reliability
- Make maintenance easier
Understanding Python Try Except
The try-except statement is Python's primary error-handling mechanism. Think of it as a safety net for your code:
python
1try: 2 # Your potentially risky code goes here 3 result = 10 / user_input 4except: 5 # Your error handling code goes here 6 print("Something went wrong!")
Basic Structure
- try: Contains code that might raise an error
- except: Handles any errors that occur
- else: Runs if no errors occur (optional)
- finally: Always runs, regardless of errors (optional)
Step-by-Step Example of Try Except
Let's look at practical examples of error handling:
1. Basic Division Error Handling
python
1try: 2 result = 10 / 0 # This will raise a ZeroDivisionError 3except ZeroDivisionError: 4 print("Oops! You can't divide by zero.")
2. Handling Multiple Error Types
python
1try: 2 number = int(input("Enter a number: ")) 3 result = 10 / number 4except ValueError: 5 print("Please enter a valid number!") 6except ZeroDivisionError: 7 print("Division by zero is not allowed!")
3. Using Try-Except with Files
python
1try: 2 with open("data.txt", "r") as file: 3 content = file.read() 4except FileNotFoundError: 5 print("File not found. Please check the path.") 6finally: 7 print("File operation attempted.")
Advanced Error Handling Techniques
1. Capturing Error Details
python
1try: 2 value = int("hello") 3except ValueError as error: 4 print(f"Error details: {error}") 5 # Outputs: Error details: invalid literal for int() with base 10: 'hello'
2. Using Try-Except-Else-Finally
python
1try: 2 number = int(input("Enter a number: ")) 3except ValueError: 4 print("Invalid input!") 5else: 6 print(f"You entered: {number}") 7finally: 8 print("Operation completed!")
3. Custom Exception Handling
python
1class CustomError(Exception):
2 pass
3
4try:
5 raise CustomError("This is a custom error")
6except CustomError as e:
7 print(f"Caught custom error: {e}")
Common Beginner Mistakes with Try Except
1. Catching All Exceptions
Bad Practice:
python
1try: 2 # Some code 3 risky_operation() 4except: # Too broad! 5 print("An error occurred")
Good Practice:
python
1try: 2 risky_operation() 3except ValueError: 4 print("Invalid value provided") 5except ZeroDivisionError: 6 print("Division by zero detected")
2. Ignoring Exception Details
Bad Practice:
python
1try: 2 process_data() 3except Exception: 4 pass # Silent failure!
Good Practice:
python
1try: 2 process_data() 3except Exception as e: 4 logging.error(f"Error processing data: {e}")
Real-World Applications
1. File Operations
python
1def read_config_file(filename):
2 try:
3 with open(filename, 'r') as file:
4 return file.read()
5 except FileNotFoundError:
6 print(f"Config file {filename} not found")
7 return None
8 except PermissionError:
9 print("Permission denied accessing file")
10 return None
2. API Requests
python
1import requests
2
3def fetch_data(url):
4 try:
5 response = requests.get(url)
6 response.raise_for_status()
7 return response.json()
8 except requests.RequestException as e:
9 print(f"API request failed: {e}")
10 return None
3. Database Operations
python
1def save_to_database(data):
2 try:
3 db.connect()
4 db.save(data)
5 except DatabaseError as e:
6 print(f"Database error: {e}")
7 finally:
8 db.disconnect()
Best Practices for Error Handling
Be Specific with Exceptions
- Catch specific exceptions rather than using bare except
- Handle different errors differently
- Log error details for debugging
Use Context Managers
- Implement proper resource cleanup
- Utilize the 'with' statement
- Handle cleanup in finally blocks
Implement Proper Logging
- Log errors with appropriate severity levels
- Include relevant context in log messages
- Use structured logging when possible
Conclusion
Mastering Python's try-except mechanism is crucial for writing robust code. Remember:
- Start with specific exceptions
- Always include error details
- Use proper cleanup mechanisms
- Implement appropriate logging
- Test error scenarios thoroughly
Want to Learn More?
Check out these related resources: