Moving from CRA to Next.js: SSR and SEO Mastery
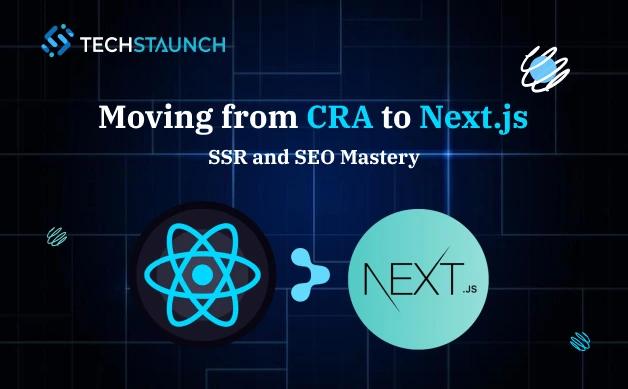
A Step-by-Step Guide to Future-Proof Your React Projects
Why Migrate to Next.js?
Create React App (CRA) was deprecated in February 2025 due to outdated tooling, lack of maintenance, and compatibility issues with modern React features like Server Components and Concurrent Mode . Next.js has emerged as the leading replacement, offering:
- Server-Side Rendering (SSR) and Static Site Generation (SSG) for SEO.
- Auto-optimizations (code-splitting, ISR, image optimization).
- Full-stack capabilities (API routes, routing, state management).
The Tooling Landscape: Why Next.js?
Next.js addresses CRA’s limitations by:
- Replacing Webpack with Vite’s esbuild for 70% faster builds .
- Native SSR/SSG to improve SEO and initial load times .
- Built-in API routes for serverless functions.
- React Server Components support (React 19+) .
Step 1: Create a New Next.js Project
Generate a Next.js App:
bash
1npx create-next-app@latest my-next-app 2cd my-next-app
Copy CRA’s Source Code:
- Replace
app/
in Next.js with your CRAsrc/
folder. - Move
public/
orassets/
to the Next.js root.
- Replace
Step 2: Update Dependencies
Remove CRA-Specific Packages:
bash
1npm uninstall react-scripts
Install Next.js Plugins:
bash
1npm install next react react-dom
Merge
package.json
:- Copy dependencies from CRA to Next.js, excluding
eslint-config-react-app
.
- Copy dependencies from CRA to Next.js, excluding
Step 3: Configure Next.js
Create
next.config.js
:javascript
1/** @type {import('next').NextConfig} */ 2const nextConfig = { 3 reactStrictMode: true, 4 images: { 5 domains: ['your-api-domain.com'], 6 }, 7} 8export default nextConfig
Update Scripts:
json
1"scripts": { 2 "dev": "next dev", 3 "build": "next build", 4 "start": "next start", 5 "lint": "next lint" 6}
Step 4: Migrate Routing
Replace CRA’s
react-router
with Next.js File-Based Routing:- Move pages to
app/
orpages/
(e.g.,pages/about.js
). Use
redirects()
innext.config.js
for legacy routes:javascript
1module.exports = { 2 redirects: () => [ 3 { source: '/old-path', destination: '/new-path', permanent: false } 4 ] 5}
- Move pages to
Step 5: Implement SSR/SSG
Server-Side Rendering (SSR):
javascript
1// pages/post.js 2export async function getServerSideProps() { 3 const data = await fetch('https://api.example.com/data'); 4 return { props: { data } }; 5}
Static Site Generation (SSG):
javascript
1export async function getStaticProps() { 2 return { props: { data } }; 3}
Step 6: Handle Environment Variables
- Rename
.env
files to.env.local
(Next.js format). - Access variables via
process.env.NEXT_PUBLIC_API_KEY
.
Step 7: Optimize Performance
Use Next.js Image Component:
jsx
1import Image from 'next/image'; 2<Image src="/logo.png" alt="Logo" width={500} height={250} />
Enable Incremental Static Regeneration (ISR):
javascript
1export async function getStaticProps() { 2 return { 3 props: { data }, 4 revalidate: 60 // Regenerate every 60 seconds 5 }; 6}
Step 8: Migrate API Routes
Create API Routes:
bash
1mkdir pages/api
Move Backend Logic:
javascript
1// pages/api/users.js 2export default function handler(req, res) { 3 res.status(200).json({ name: 'John Doe' }); 4}
Step 9: Test Incrementally
- Verify SSR: Check if pages render on the server.
- Test Routing: Ensure all legacy paths redirect correctly.
- Audit SEO: Use Google Lighthouse for SEO scores.