Migrating CRA to Vite: A Step-by-Step Guide
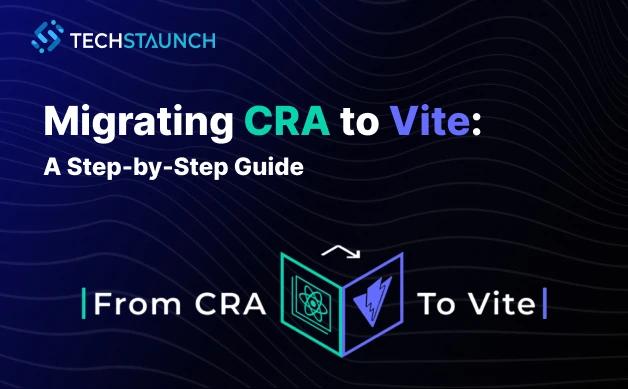
How to Future-Proof Your React Projects with Vite’s Speed and Flexibility
Why Migrate to Vite
Vite offers:
- 70% faster builds than CRA (esbuild vs. Webpack).
- Instant HMR updates without page reloads.
- Zero-config simplicity for small-to-medium apps.
- Native support for modern JS features (Top-Level Await, ES modules).
Prerequisites
- Node.js (v18+ recommended).
- Existing CRA project with React/TypeScript.
- Backup your project (Git branch or cloud storage).
Step 1: Create a New Vite Project
Generate a Vite Template:
bash
1npm create vite@latest my-vite-app --template react 2cd my-vite-app
This creates a minimal Vite setup with React.
Copy Source Code:
- Replace
src/
in the Vite project with your CRAsrc/
folder. - Move
public/
orassets/
to the Vite root.
- Replace
Step 2: Update Dependencies
Remove CRA-Specific Packages:
bash
1npm uninstall react-scripts
Install Vite Plugins:
bash
1npm install vite @vitejs/plugin-react-swc vite-tsconfig-paths vite-plugin-svgr
(Use
@vitejs/plugin-react
for JSX) .Copy Dependencies:
- Merge
dependencies
anddevDependencies
from your CRApackage.json
into the Vite project. - Remove CRA-specific entries (e.g.,
eslint-config-react-app
).
- Merge
Step 3: Configure Vite
Create
vite.config.ts
:typescript
1import { defineConfig } from 'vite' 2import react from '@vitejs/plugin-react-swc' 3 4export default defineConfig({ 5 base: '/', 6 plugins: [react()], 7 resolve: { 8 alias: { 9 '@': '/src', 10 }, 11 }, 12})
(Adds
@
alias forsrc/
) .Update Scripts:
json
1"scripts": { 2 "dev": "vite", 3 "build": "tsc && vite build", 4 "serve": "vite preview", 5 "test": "vitest", 6 "lint": "eslint src --ext ts,tsx" 7}
Step 4: Fix TypeScript/JSX Configurations
Update
tsconfig.json
:json
1{ 2 "compilerOptions": { 3 "target": "ESNext", 4 "lib": ["dom", "esnext"], 5 "jsx": "react-jsx", 6 "module": "esnext", 7 "moduleResolution": "node", 8 "resolveJsonModule": true, 9 "strict": true, 10 "esModuleInterop": true, 11 "skipLibCheck": true 12 }, 13 "include": ["src"] 14}
Add
vite-env.d.ts
:typescript
1/// <reference types="vite/client" />
Step 5: Migrate Testing (Optional)
Switch from Jest to Vitest:
bash
1npm install -D jsdom vitest @vitest/coverage-v8
Update
vite.config.ts
:typescript
1export default defineConfig({ 2 test: { 3 globals: true, 4 environment: 'jsdom', 5 coverage: { 6 reporter: ['text', 'html'], 7 }, 8 }, 9})
Add Test Scripts:
json
1"test": "vitest", 2"test:coverage": "vitest run --coverage"
Step 6: Handle Environment Variables
- Rename
.env
files to.env.vite
(Vite’s format). - Access variables via
import.meta.env.VITE_API_KEY
.
Step 7: Configure Proxy Server (If Needed)
Add
proxy
tovite.config.ts
:typescript
1server: { 2 proxy: { 3 '/api': 'http://localhost:3000', 4 }, 5}
Step 8: Enable Gzip Compression (Optional)
Install
vite-plugin-compression
:bash
1npm install vite-plugin-compression -D
Update
vite.config.ts
:typescript
1import compression from 'vite-plugin-compression' 2 3export default defineConfig({ 4 plugins: [react(), compression()], 5})
Step 9: Final Cleanup
- Delete Unused Files:
- Remove
node_modules/.cache
,build/
, andpublic/*.html
(CRA artifacts).
- Remove
- Test Incrementally:
- Verify HMR, routing, and API calls.
Migrating to Vite can significantly boost your app’s performance and developer experience. If your team needs help with this migration or any development, reach out to us! We specialize in modernizing legacy apps and building scalable React solutions.