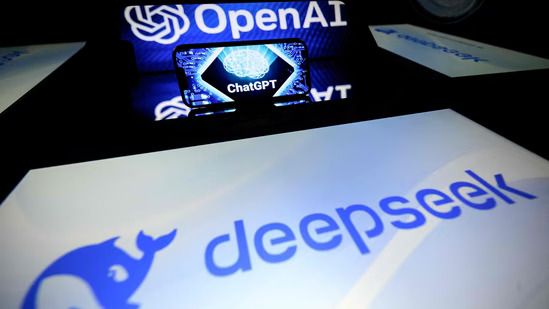
Introduction
Looking to harness the power of DeepSeek in your Node.js applications? You're in the right place. DeepSeek offers powerful AI capabilities, and the best part? Its API works just like OpenAI's, making integration a breeze. Whether you're building a chatbot or working on content generation, this guide will walk you through the process step by step.
Why Choose DeepSeek with Node.js?
Before we dive in, here's why this combination makes sense:
- API Compatibility: Works seamlessly with OpenAI's SDK
- Cost-Effective: Better pricing compared to alternatives
- Powerful Features: Advanced AI capabilities for various use cases
- Easy Integration: Simple setup process with Node.js
Prerequisites
Let's make sure you have everything ready:
- ✅ Node.js installed on your system
- ✅ A DeepSeek API key (grab one from their platform)
- ✅ Basic JavaScript knowledge
- ✅ Your favorite code editor
Step-by-Step Integration
1. Set Up Your Project
First, let's create your project structure:
bash
1mkdir deepseek-project 2cd deepseek-project 3npm init -y
2. Install Dependencies
Get the OpenAI SDK installed:
bash
1npm install openai
3. Configure DeepSeek
Create a config file that sets up the connection:
javascript
1// config.js 2const OpenAI = require('openai'); 3 4const openai = new OpenAI({ 5 apiKey: process.env.DEEPSEEK_API_KEY, // Use environment variables! 6 baseURL: 'https://api.deepseek.com', 7}); 8 9module.exports = openai;
4. Create Your First Integration
Let's write a simple script to test things out:
javascript
5. Test Your Integration
Run your script:
bash
1node app.js
Common Errors & Troubleshooting
API Key Issues
If you see authentication errors:
javascript
1// Check your environment variable 2console.log('API Key:', process.env.DEEPSEEK_API_KEY?.slice(0, 5) + '...');
Connection Problems
For network-related issues:
javascript
1// Add timeout and retry logic 2const openai = new OpenAI({ 3 timeout: 30000, // 30 seconds 4 maxRetries: 3 5});
Best Practices
1. Secure Your Credentials
Never hardcode API keys:
javascript
1// Use dotenv for environment variables 2require('dotenv').config();
2. Handle Rate Limits
Implement proper error handling:
javascript
1try { 2 // Your API call 3} catch (error) { 4 if (error.status === 429) { 5 // Handle rate limit 6 await delay(1000); // Wait before retry 7 } 8}
3. Optimize Performance
Cache responses when possible:
javascript
1const NodeCache = require('node-cache'); 2const cache = new NodeCache({ stdTTL: 600 }); // 10 minutes
Migration Tips
Coming from OpenAI? Here's what changes:
Update the base URL:
javascript
1baseURL: 'https://api.deepseek.com'
Update model names:
javascript
1model: 'deepseek-chat' // Instead of 'gpt-3.5-turbo'
Wrapping Up
You've now got DeepSeek integrated with your Node.js application! Remember to:
- Keep your API keys secure
- Monitor your usage
- Implement proper error handling
- Stay updated with DeepSeek's documentation
Need More Help?
Check out these resources: